Understanding Python String Indexing: A Comprehensive Tutorial
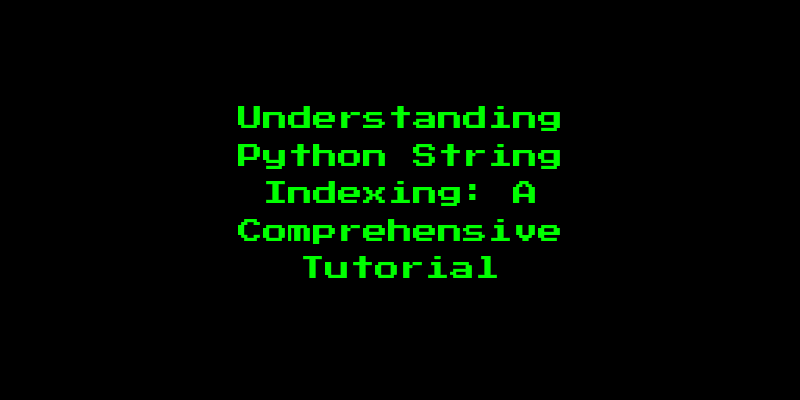
Introduction
Python string indexing is a powerful feature that allows you to access and manipulate individual characters within a string. In this tutorial, we will explore the core concepts of string indexing, syntax, usage, common pitfalls, best practices, practical examples, and conclusion.
Core Concepts
String indexing in Python refers to the process of accessing individual characters within a string using their position or index. Each character in a string has a unique position or index number that can be used to access it. The first character in a string is at index 0, and the last character is at index -1 (the length of the string minus one).
Syntax and Usage
To access individual characters within a string using indexing, you use square brackets []
followed by the index number. For example:
string = "Hello World"
print(string[0]) # Output: H
print(string[-1]) # Output: d
You can also use negative indices to access characters from the end of the string, starting with -1 for the last character.
string = "Hello World"
print(string[-2]) # Output: l
Common Pitfalls (Optional)
One common pitfall when working with string indexing is trying to access an index that is out of range. For example, if you try to access the character at index 10 in a string that only has 5 characters, you will get an error.
string = "Hello"
print(string[10]) # Error: IndexError: list index out of range
To avoid this error, always check the length of the string before trying to access individual characters using indexing.
Best Practices
When working with strings in Python, it is generally considered best practice to use slicing instead of indexing whenever possible. Slicing allows you to access a range of characters within a string, rather than just a single character. For example:
string = "Hello World"
print(string[0:5]) # Output: Hello
print(string[-6:-1]) # Output: World
This can be more efficient and readable code than using indexing for each individual character.
Practical Examples
One practical example of string indexing is parsing a string to extract specific information. For example, if you have a string that contains the name, age, and gender of a person, you could use indexing to extract just their name or age.
string = "John Smith, 35, male"
name = string[0:8] # Output: John Smith
age = int(string[-4]) # Output: 35
gender = string[-1:] # Output: male
print("Name:", name)
print("Age:", age)
print("Gender:", gender)
Conclusion
String indexing is a powerful feature in Python that allows you to access and manipulate individual characters within a string. By understanding the core concepts, syntax, and usage of string indexing, you can write more efficient and readable code when working with strings in Python.